How to use flutter_localizations in FlutLab
1. Open your Profile and press the “+ Project” button.
2. Create a new project with “CodeBase: Stateless Hello World”.
3. Open pubspec.yaml file and add the packages as a dependency:
flutter_localizations:
sdk: flutter
intl: ^0.17.0
4. Also, in the pubspec.yaml
file, enable the generate
flag:
generate: true
5. Press the “pub get” button.
6. Wait for the result - “Process finished successfully”
7. Let’s create an l10n.yaml
file inside the root folder. Click the “Create file” icon (look at the screenshots below):
8. File was successfully created. Add these lines inside it:
arb-dir: lib/l10n
template-arb-file: app_en.arb
output-localization-file: app_localizations.dart
9. Create l10n
folder inside lib
folder.
10. Create app_en.arb
file inside lib/l10n
folder.
11. Add code there
{
"helloWorld": "Hello World!",
"@helloWorld": {
"description": "The conventional newborn programmer greeting"
}
}
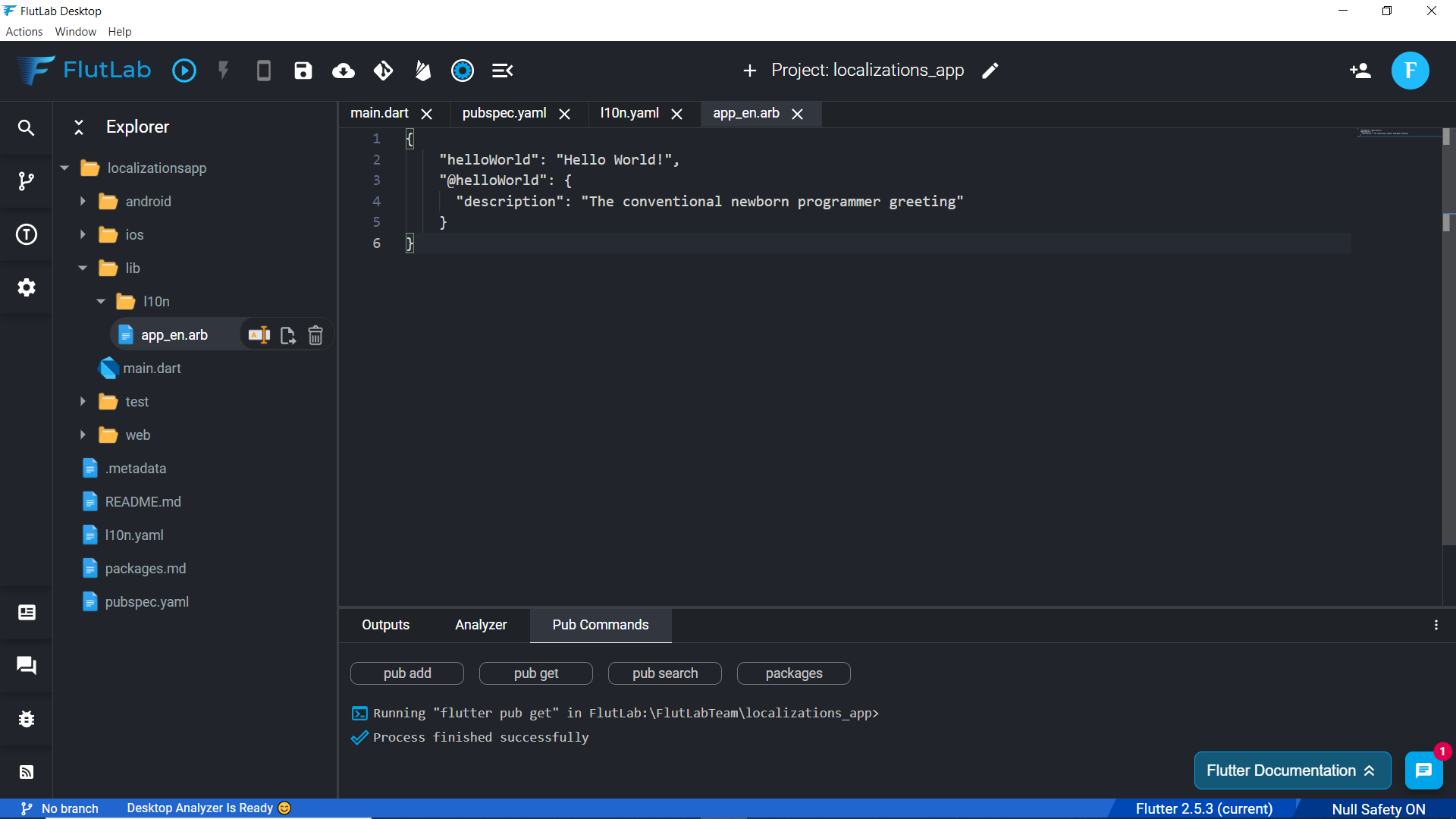
12. Create app_es.arb
file inside lib/l10n
folder.
13. Add code there
{ "helloWorld": "¡Hola Mundo!" }
14. Press the “Build Project” button and run your app.
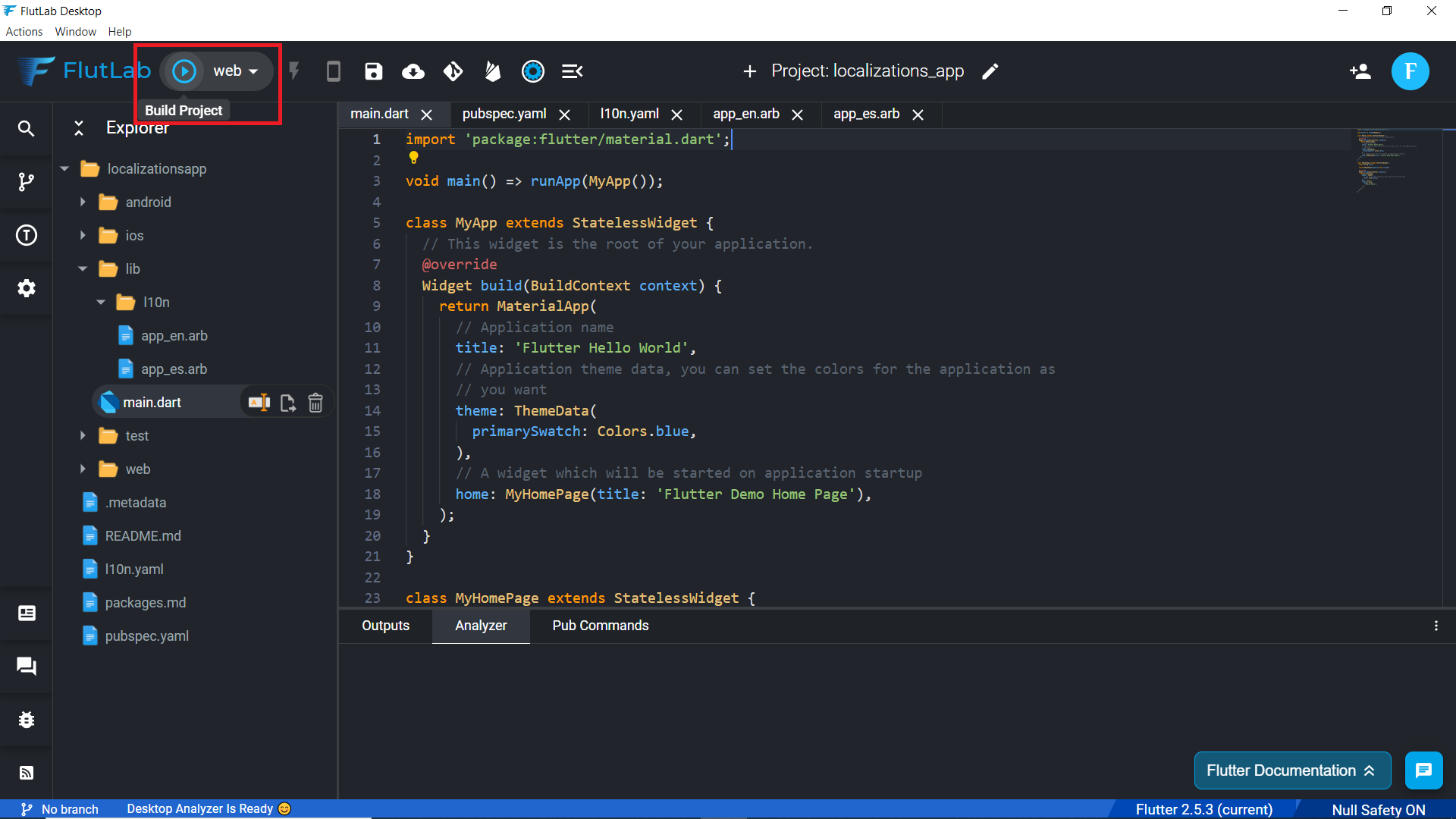
15. So that codegen takes place. You should see generated files in /.dart_tool/flutter_gen/gen_l10n
.
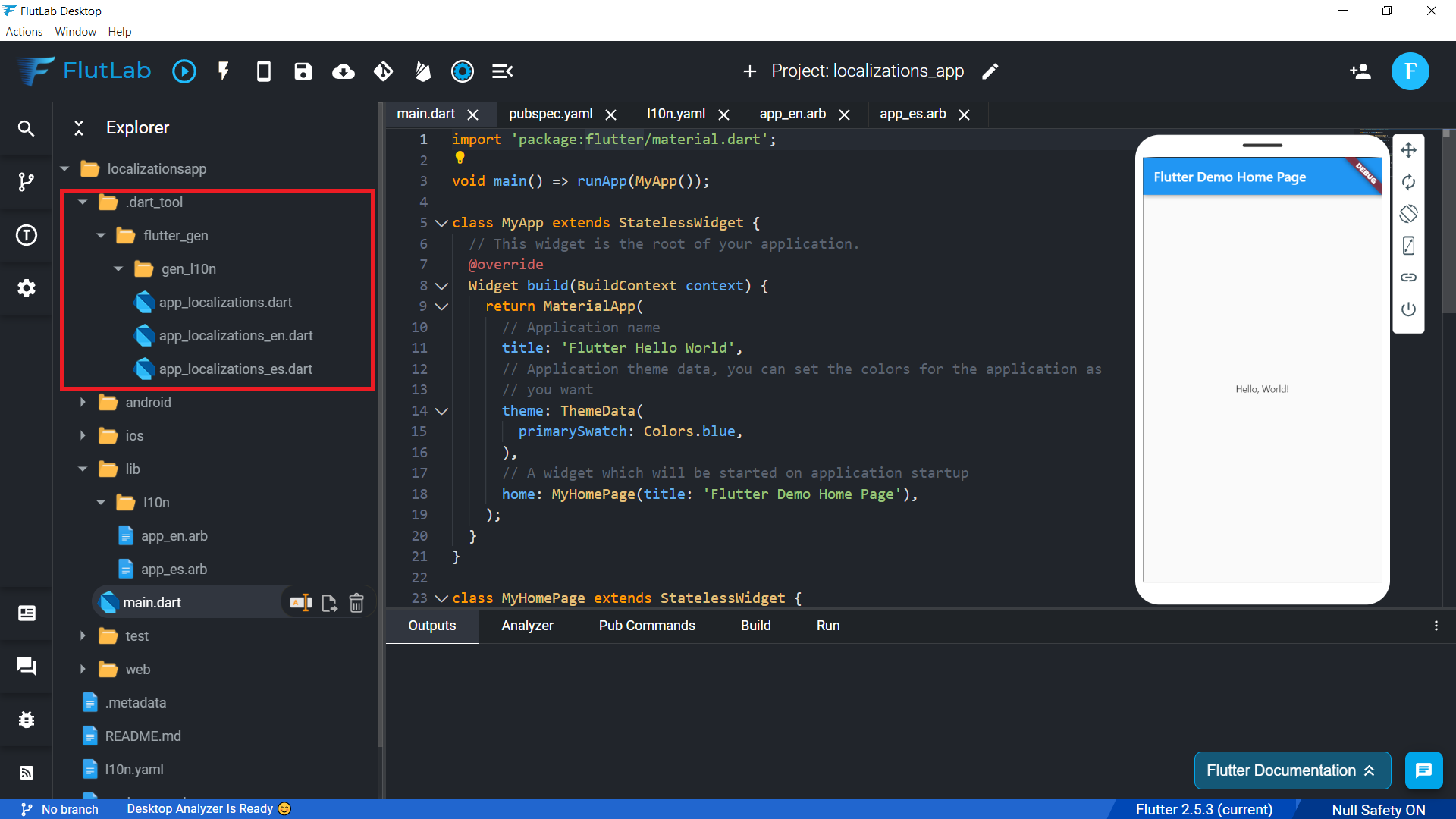
16. Add the import to your main.dart
file:
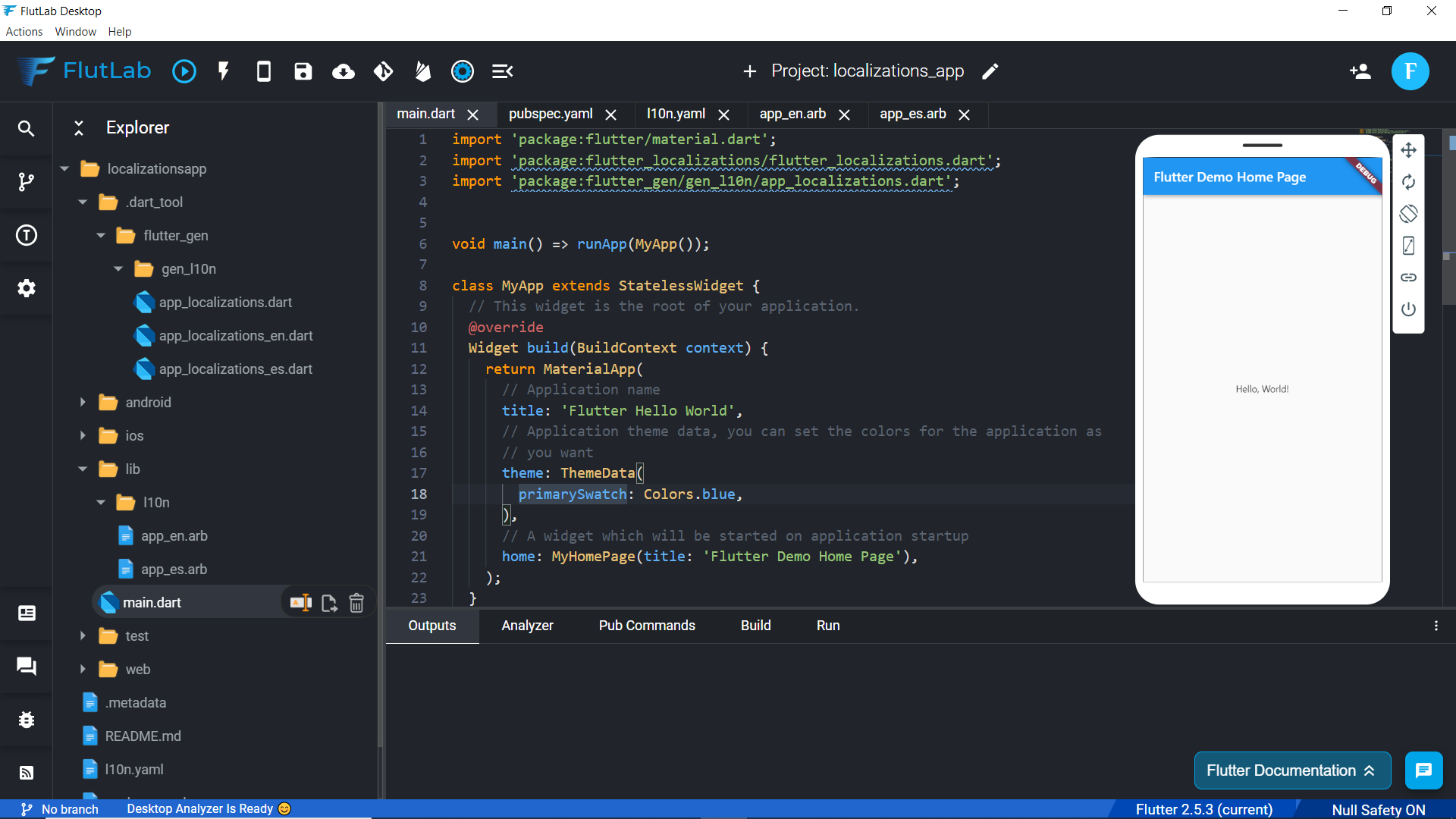
17. In the main.dart
file find line 14 and add this code:
locale: Locale('es', ''),
localizationsDelegates: [
AppLocalizations.delegate, // Add this line
GlobalMaterialLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
GlobalCupertinoLocalizations.delegate,
],
supportedLocales: [
Locale('en', ''), // English, no country code
Locale('es', ''), // Spanish, no country code
],
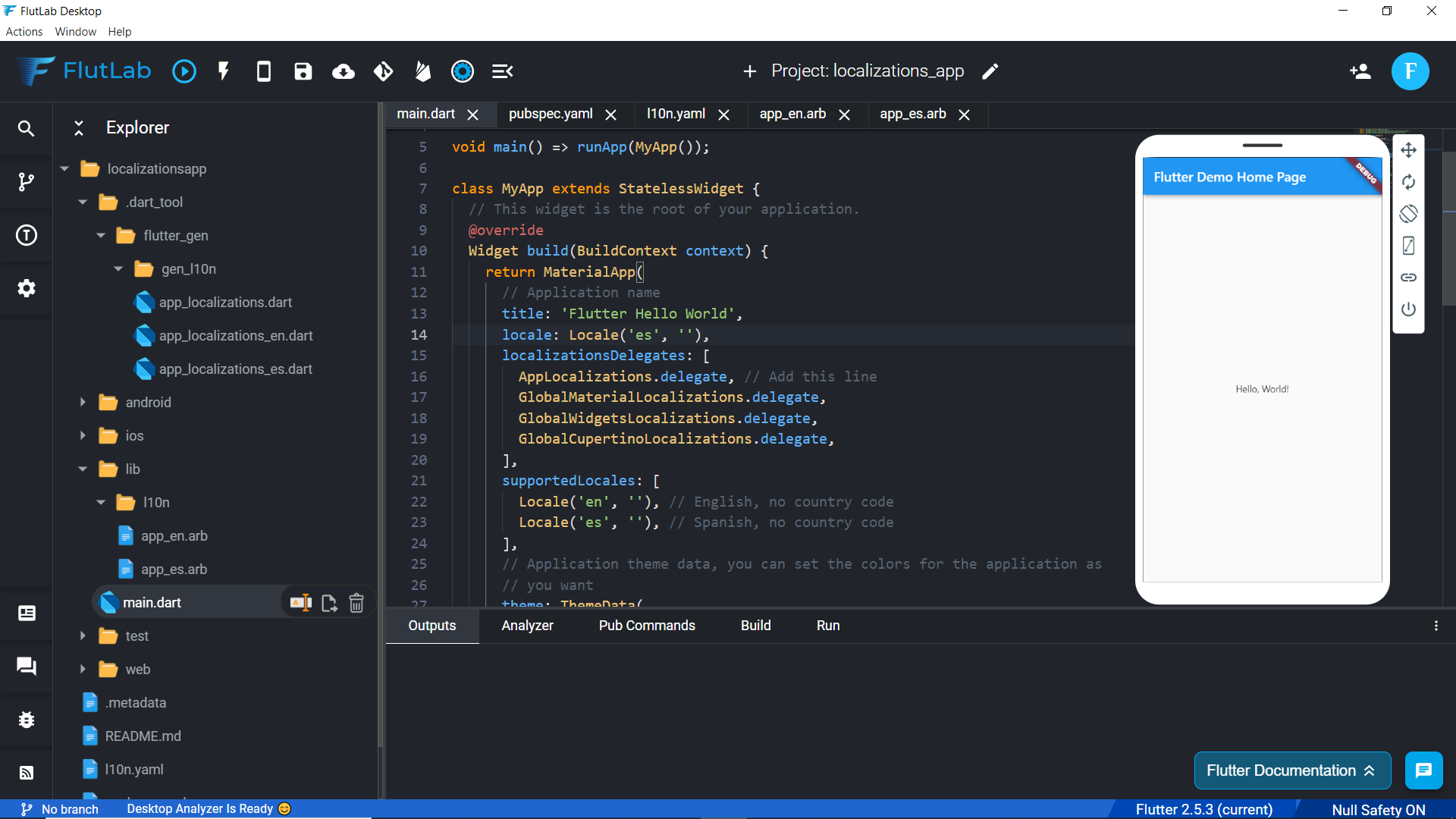
18. Replace this fragment of code (line 49)
Text('Hello, World!'),
with this one
Text(AppLocalizations.of(context)!.helloWorld),
19 Press the “Build Project” button with web-build.
20. If you will open app_localizations.dart
file, you can find generated localizationsDelegates
and supportedLocales
list:
21. So, this allows you to write more concise code and use the generated localizationsDelegates
and supportedLocales
list instead of providing them manually.
localizationsDelegates: AppLocalizations.localizationsDelegates,
supportedLocales: AppLocalizations.supportedLocales,
Read More
https://docs.flutter.dev/development/accessibility-and-localization/internationalization
Supported languages
af
- Afrikaansam
- Amharicar
- Arabicas
- Assameseaz
- Azerbaijanibe
- Belarusianbg
- Bulgarianbn
- Bengali Banglabs
- Bosnianca
- Catalan Valenciancs
- Czechda
- Danishde
- German (plus one country variation)el
- Modern Greeken
- English (plus 8 country variations)es
- Spanish Castilian (plus 20 country variations)et
- Estonianeu
- Basquefa
- Persianfi
- Finnishfil
- Filipino Pilipinofr
- French (plus one country variation)gl
- Galiciangsw
- Swiss German Alemannic Alsatiangu
- Gujaratihe
- Hebrewhi
- Hindihr
- Croatianhu
- Hungarianhy
- Armenianid
- Indonesianis
- Icelandicit
- Italianja
- Japaneseka
- Georgiankk
- Kazakhkm
- Khmer Central Khmerkn
- Kannadako
- Koreanky
- Kirghiz Kyrgyzlo
- Laolt
- Lithuanianlv
- Latvianmk
- Macedonianml
- Malayalammn
- Mongolianmr
- Marathims
- Malaymy
- Burmesenb
- Norwegian Bokmålne
- Nepalinl
- Dutch Flemishno
- Norwegianor
- Oriyapa
- Panjabi Punjabipl
- Polishps
- Pushto Pashtopt
- Portuguese (plus one country variation)ro
- Romanian Moldavian Moldovanru
- Russiansi
- Sinhala Sinhalesesk
- Slovaksl
- Sloveniansq
- Albaniansr
- Serbian (plus 2 scripts)sv
- Swedishsw
- Swahilita
- Tamilte
- Teluguth
- Thaitl
- Tagalogtr
- Turkishuk
- Ukrainianur
- Urduuz
- Uzbekvi
- Vietnamesezh
- Chinese (plus 2 country variations and 2 scripts)zu
- Zulu